Writing a Plugin for Linux
Write your own plugin in Python or Shell and monitor the data you need, the way you want. Set thresholds to individual attributes and be alerted if the set values exceed.
Ensure the latest version of the Site24x7 Linux Monitoring agent is installed in your server.
- Format of plugin output
- Steps to write a plugin
- Multiple configurations in a single plugin script
- Edit/modify added plugin monitor
- Edit a plugin template
- Sample plugin for Python - Monitor the open files in a Linux server
- Sample plugin for Shell - Monitor the number of files and directories in a Linux server
- Licensing
- Troubleshooting Tips
Format of Plugin Output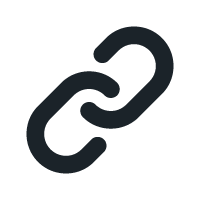
The plugin script should return a JSON object. Provide only one level of JSON data as key-value pair. The JSON data to be posted to the Site24x7 server should be in the following format:
{ "plugin_version" : 1, "heartbeat_required" : true, "cpu" : 42.7, "memory" : 65.8, "network" : 156, "units" : { "cpu" : "%" , "memory" : "%" , "network" : "MB" } }
If you want to see a tabular view under the plugin monitor, the JSON format can be a nested JSON with two levels. However, for the plugin to be registered successfully, at least one parent integer attribute needs to be present.
For example, here is the format of the JSON data that can be posted to Site24x7 to get a tabular view:
{
"plugin_version": 1,
"heartbeat_required": true,
"units": {
"Tablespace_Details": {
"Tablespace_Size": "mb",
"Used_Percent": "%",
"Used_Space": "mb"
}
},
"Tablespace_Details": [
{
"name": "SDSCLN",
"Used_Space": 103.93,
"Tablespace_Size": 32767.98,
"Used_Percent": 0.31,
"TB_Status": 1,
"status": 1
},
{
"name": "SDSCLNCDR",
"Used_Space": 1,
"Tablespace_Size": 32767.98,
"Used_Percent": 0.003,
"TB_Status": 1,
"status": 1
}
],
"Tablespace_Count": 2,
}
- plugin_version: Denotes the version number of the plugin
- This is a mandatory field
- When there is any change in the plugin_version, the Site24x7 data centre updates the template and creates a new template for that version
- The default value is 1. If any change is done in the plugin, user must increment the plugin_version by 1
- plugin_version should be whole numbers only. E.g. 1,2,3...
- units: Units of the attributes monitored
- This is an optional field
- It is a key value pair with keys as the name of the attributes monitored in the plugin and the values as their respective units
- For example, while measuring the cpu utilization percentage in a plugin
units : {'cpu':'%'}
- status: Denotes the availability of the monitor.
- The value can either be:
- 1, which means the status is Up and metrics are collected successfully, or
- 0, indicating the status is Down and metrics cannot be collected due to a script error or failure to connect to the remote server or API.
- This is a reserved key and should not be used for any other functions in the plugin script.
- Capture the status field as an exception and set it as 0 in the custom script. This ensures that the monitor goes down if there is an error while executing the plugin. The status field is set to 0 by default.
- Along with the status, you can configure the msg field to capture any error message while executing the plugin.
- The value can either be:
- msg: Messages for the monitor
- This is an optional field
- The configured error message would show up in the Site24x7 web client if the monitor is down
- This attribute should be placed parallel to the data attribute
Steps to Write a Plugin 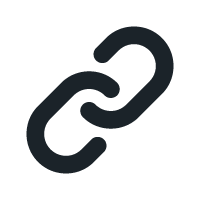
- Download and install the latest version of the Site24x7 Linux agent in your server.
- Go to Server > Plugin Integrations > Write your own Plugin.
- Download the sample code from any of the available formats: Shell or Python script.
- In the downloaded sample script, find the Method named getData( ) and edit this with your own logic to return the required performance data.
Note- In the sample code, please do not delete the #Mandatory Fields.
- Do not use the reserved keys in the custom script while writing the plugin. See the list of all reserved keys.
- You can validate the output of your plugin script by executing it manually in the terminal.
Example: " python mysql.py"
- Create a folder in the /opt/site24x7/monagent/plugins/<foldername> and place your plugin script file in this folder. Make sure the name of the file and the folder name are identical. For eg., If you are writing a plugin for Apache, the plugin script file should be apache.py and the folder name should be Apache.
NoteFor the Docker agent, go to the respective container by executing the following command:
docker exec -it site24x7-agent /bin/bash
In the next agent data collection, the plugin will be discovered and marked up for monitoring. You can see the monitor under Server > Plugin Integrations. The plugin monitor will also be listed under the respective server monitor's Plugins tab (Server > Server Monitor > Servers > click on the desired server monitor > Plugins). You can also set up threshold profiles and be alerted when the configured value exceeds.
All plugin logs are captured in the file plugins.txt under agent logs directory that can be accessed to troubleshoot plugins. Find the logs file under opt/site24x7/monagent/logs/details
Multiple Configurations in a Single Plugin Script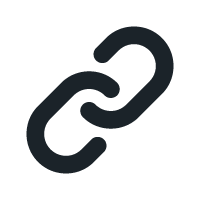
When there is more than one configuration for executing a plugin, creating a new monitor for every configuration (with different names) can turn time consuming and laborious. To resolve this, Site24x7 Plugin Monitoring supports executing multiple configurations using a single plugin script.
- Example 1: Monitor MySQL instances running on same hosts using a single plugin
- Example 2: Monitor the file or directory count of more than one folder using a single plugin
Example 1:
Consider having multiple MySQL instances, say production_master and production_slave, running in your environment. Instead of using multiple MySQL plugins, you can define all the configurations and execute them all using a single plugin script file. Before getting started, ensure the required dependency modules are installed to run the plugin. Then, follow the steps below:
- Create a folder mysql and place the plugin script file mysql.py inside the folder.
- Create a configuration file named after the plugin, mysql.cfg, and place it inside the folder mysql. Define the configurations in the mysql.cfg file as below:
[production_master]
hostname='193.167.1.0'
port = 3306
username = 'test'
password = 'test'
[production_slave]
hostname='194.168.2.0'
port = 3306
username = 'test'
password = 'test' - Add the following piece of code in the main function of the mysql.py script file to read the arguments configured in the mysql.cfg file. Ignore if the code is already present in the plugin script file.
Note
Ensure the indentation is right in the plugin script file, after copy-pasting the below code.
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--host', help="mysql host",type=str)
parser.add_argument('--port', help ="mysql port",type=str)
parser.add_argument('--username', help='mysql username', type=str)
parser.add_argument('--password', help='mysql password', type=str)
args = parser.parse_args()
if args.host:
MYSQL_HOST = args.host
if args.port:
MYSQL_PORT = args.port
if args.username:
MYSQL_USERNAME = args.username
if args.password:
MYSQL_PASSWORD = args.password
- Check if the configurations defined in mysql.cfg is working as expected by executing the following commands:
/usr/bin/python mysql.py --host=192.168.1.0 --port=3306 --username=test --password=test
/usr/bin/python mysql.py --host=192.168.2.0 --port=3306 --username=test --password=testNoteEnsure the MySQL performance metrics are returned as JSON output. If any error occurs, fix the same and proceed to the next step.
- Copy-paste the mysql folder to the agent's plugin directory /opt/site24x7/monagent/plugins/. In the next data collection, the Linux agent will encrypt the values in the .cfg file, execute the mysql.py file, and add a new plugin monitor for each configuration (given in mysql.cfg file) in the Site24x7 web client. A sample configuration file after encryption would look like:
[production_master]
encrypted.password = ojhVVrdlXKdW/s3YPJuhzCKhBcQF8PIUThEvqGeUijI=
[production_slave]
encrypted.password = XzIGEreeTblUFrvUJWDcIArzN5qi0qHFxZVUt1KqjyY=
In the Site24x7 web client, three plugin monitors will be added for monitoring. The display name of the plugin monitor in the Site24x7 web client will be in the format <plugin_script_name>-<section_name_in_the_.cfg_file>-<server-hostname>.
Eg: In the sample configuration above, the monitor display name for one of the monitors will be mysql.py-production_master-<server-hostname>
Encryption of Plugin Configuration Details:
By default, the Linux server monitoring agent will encrypt the field 'password' given in the plugin configuration file. Once the plugin is executed, the configuration file will be updated as follows:
[production_master]
host=192.168.1.0
port = 3306
username = 'test'
encrypted.password = ojhVVrdlXKdW/s3YPJuhzCKhBcQF8PIUThEvqGeUijI=
[production_slave]
host=192.168.2.0
port = 3306
username = 'test'
encrypted.password = XzIGEreeTblUFrvUJWDcIArzN5qi0qHFxZVUt1KqjyY=
If any other field needs to be encrypted along with the password, configure it in the global_configurations section of mysql.cfg file.
[global_configurations]
keys_to_encrypt=password,username
[production_master]
host=192.168.2.0
port = 3306
username = 'test'
password = 'test'
[production_slave]
host=192.168.2.0
port = 3306
username = 'test'
password = 'test'
Edit the Configuration Details:
In case there is a change in any of the values provided in the configuration file, you can edit it by removing the 'encrypted.' parameter from the defined keys.
For example, if there is a change in the password of [production_master] section, edit the mysql.cfg file by removing the parameter 'encrypted.' from the key encrypted.passsword and entering the new password. During the next consecutive data collection, the agent will detect the configuration change, encrypt the new values, and update the file accordingly.
Example 2:
Consider monitoring the file or directory count of more than one folder. Instead of using multiple plugins, you can define all the configurations and execute them all using a single plugin script file. To do so,
- Create a folder check_file_count.
- Download the files check_file_count.cfg and check_file_count.py from our GitHub repository and place it under the check_file_count folder.
- Edit the check_file_count.cfg file to change the configurations accordingly.
Note
By default, two sets of folder configurations are already configured in the file. Create a new section to extend it.
- Configure thresholds to be notified when the file or directory count exceeds the given value. In the check_file_count.cfg file, configure thresholds as follows:
/usr/bin/python check_file_count.py --folder_name=/ --file_count_threshold=20 --directory_count_threshold=10
/usr/bin/python check_file_count.py --folder_name=/opt --file_count_threshold=20 - Copy-paste the check_file_count folder to the agent's plugin directory /opt/site24x7/monagent/plugins/. In the next data collection, the Linux agent will execute the check_file_count.py file and add a new plugin monitor for each configuration (given in check_file_count.cfg file) in the Site24x7 web client.
Edit/Modify Added Plugin Monitor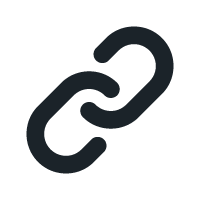
- Once a plugin monitor is successfully added, you can go to Server > Plugins > click on the plugin monitor that you have added.
- Hover on the hamburger icon beside the display name and click on Edit.
- You can edit or replace the existing display name with a new one in the Edit Plugin Monitor page.
- Set the Poll Interval for data collection, ranging from one minute to one day.
- Customize the Script Execution Timeout for your plugin scripts. The default timeout value is 30 seconds.
- Under Advanced Configuration,
- Threshold and Availability: Retain the default threshold profile or select an existing one from the dropdown or add a new one using the (+) icon and get notified when a resource exceeds the configured threshold value.
- Notification Profile: Retain the default profile or choose an existing one or add a new one (using the + icon) and configure when and who needs to be notifed in case of downtime.
- User Alert Groups: Select an existing user group or Add a User Alert Group that needs to be alerted during an outage. The user's Admin Group will be selected by default.
- Associate with Monitor Group(s): Choose an existing Monitor Group or create a new one.
- Tags: Classify and sort related resources in your account by applying unique labels. Use Add Tag to add a new one.
- IT Automation: Select an existing automation profile or Add Automation from the dropdown. Map the condition when you want to execute that particular automation.
- Third Party Integration: Integrate Site24x7 alarms to a preferred third party service.
- Save your changes.
Edit a Plugin Template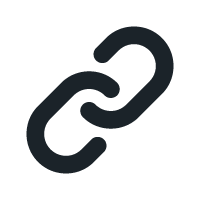
A plugin template can be edited, after adding a plugin monitor. Go to Admin > Server Monitor > Plugin Template Config > Edit Template Definition (OR) Server > Plugins > Click on the plugin monitor that has the template associated with it > Customize View.
Read on to know how to edit a template
Sample Plugin for Python - Monitor the Open Files in a Linux Server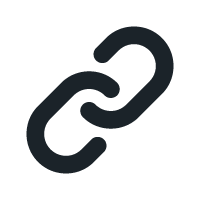
#!/usr/bin/python3
import sys,json
#if any impacting changes to this plugin kindly increment the plugin version here.
PLUGIN_VERSION = "1"
#Setting this to true will alert you when there is a communication problem while posting plugin data to server
HEARTBEAT="true"
PROC_FILE = "/proc/sys/fs/file-nr"
METRIC_UNITS = {'open_files': 'units', 'total_files': 'units'}
def metricCollector():
data = {}
data['plugin_version'] = PLUGIN_VERSION
data['heartbeat_required']=HEARTBEAT
try:
open_nr, free_nr, max = open(PROC_FILE).readline().split("\t")
open_files = int(open_nr) - int(free_nr)
data["open_files"] = open_files
data["total_files"] = int(max)
data["units"] = METRIC_UNITS
except Exception as e:
data["status"] = 0
data["msg"] = str(e)
return data
if __name__ == "__main__":
result = metricCollector()
print(json.dumps(result, indent=4, sort_keys=True))
- #!/usr/bin/python: Mention the 'shebang character' (#!) at the top of your plugin file. This will be the path of Python with which your plugin file is executed
- A Python script should return a JSON data
JSON output:
{
"open_files": 12512,
"total_files": 1620019,
"units": {
"open_files": "units",
"total_files": "units"
}
}
Data as represented in the Site24x7 dashboard:

Sample Plugin for Shell - Monitor the Number of Files & Directories in a Linux Server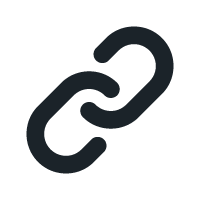
#!/bin/bash
##### Site24x7 Configuration Constants #####
PLUGIN_VERSION=1 # Set version of the plugin template used. For each plugin version, there will be a set of metrics monitored.
HEARTBEAT=false # Set if plugin has to be alerted when data is not collected for sometime
##### Plugin Input Constants #####
DIRNAME=. # Location in Which the File Count and Directory Count will be Taken
SEARCH_LEVEL=1 # Upto Which Level of Inner Directories to count the Directories and Files
##### Plugin Code #####
PLUGIN_OUTPUT=""
PLUGIN_OUTPUT=$PLUGIN_OUTPUT"plugin_version:$PLUGIN_VERSION|"
PLUGIN_OUTPUT=$PLUGIN_OUTPUT"heartbeat_required:$HEARTBEAT|"
##### Plugin Logic to get the number of files and directories
FILES=`find $DIRNAME -maxdepth $SEARCH_LEVEL -type f | wc -l`
DIRS=`find $DIRNAME/* -maxdepth $SEARCH_LEVEL -type d | wc -l`
##### Add Key and value to be monitored
##### Replace the sample keys with the metrics you want to monitor
##### Execute the commands and store the values in a variable
##### Replace the dummy values with the variables to monitor
PLUGIN_OUTPUT=$PLUGIN_OUTPUT"file_count:$FILES|"
PLUGIN_OUTPUT=$PLUGIN_OUTPUT"dir_count:$DIRS"
##### Add the units for the values to be monitored #####
UNITS="units:{"
UNITS=$UNITS"file_count-count,"
UNITS=$UNITS"dir_count-count"
UNITS=$UNITS"}"
##### Remove if not required
PLUGIN_OUTPUT="$PLUGIN_OUTPUT|$UNITS"
##### Print the data
echo "$PLUGIN_OUTPUT"
- #!/bin/bash: Mention the 'shebang character' (#!) at the top of your plugin file. This means that the script should always be run with bash
- The output will have a key-value pair, separated by a delimiter (|) from another pair
Sample JSON output:
plugin_version:1|heartbeat_required:false|file_count:18|dir_count:26|units:{file_count-count,dir_count-count}
Data as represented in the Site24x7 dashboard:
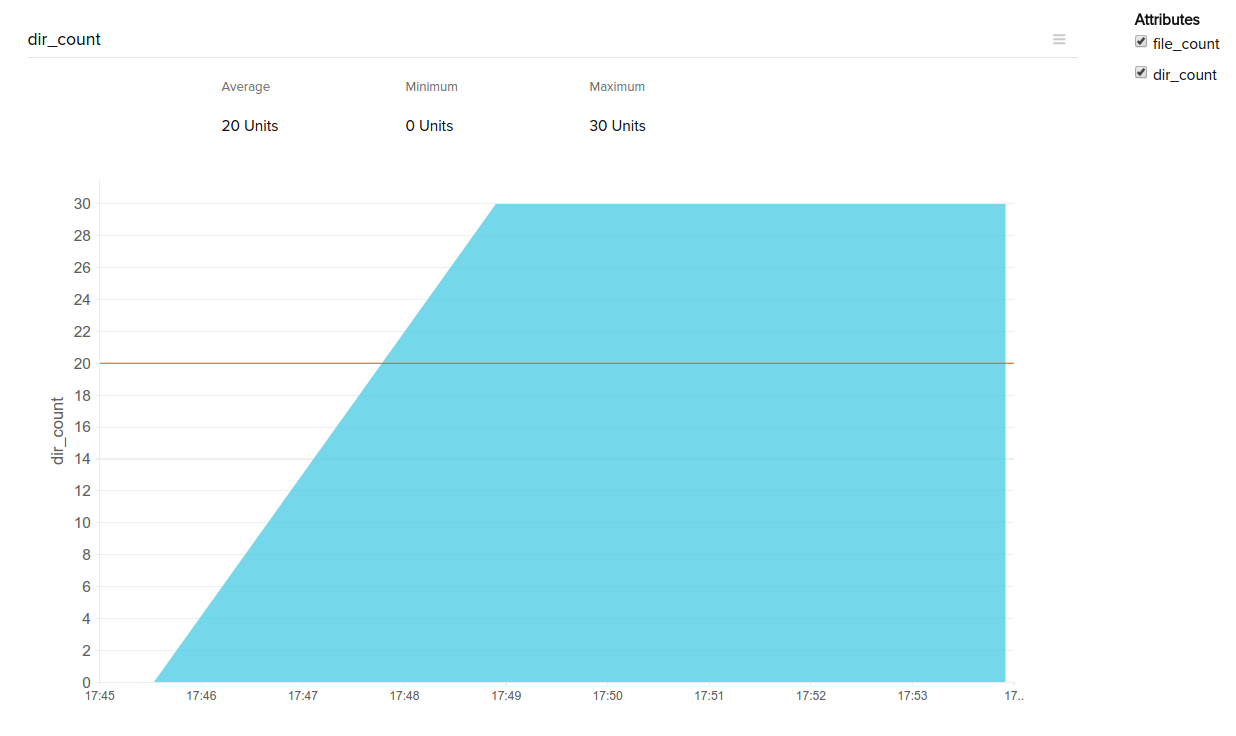
Licensing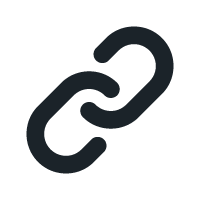
The first plugin added for a server monitor is free. After that, each plugin monitor is considered as a basic monitor.
Troubleshooting Tips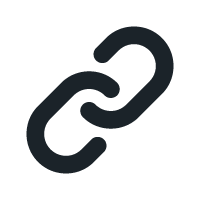
- Possible reasons why my Linux plugin is not added to my Site24x7 account
- Prerequisites to configure Linux plugins in Site24x7
- Error handling messages for plugins
- Debug a plugin monitor
- Possible steps to be performed if your plugin is in the suspended state
- What happens if I delete a plugin monitor from the Site24x7 web client?
- Run python plugin scripts in Windows servers
Difficulty in writing a plugin? Or do you have any specific requirement? Not to Worry! Post it in our Community Forum and we shall get it done for you.
Related Articles
-
On this page
- Format of Plugin Output
- Steps to Write a Plugin
- Multiple Configurations in a Single Plugin Script
- EditModify Added Plugin Monitor
- Edit a Plugin Template
- Sample Plugin for Python - Monitor the Open Files in a Linux Server
- Sample Plugin for Shell - Monitor the Number of Files Directories in a Linux Server
- Licensing
- Troubleshooting Tips