Custom instrumentation for Python applications
By default, the APM Insight Python agent captures incoming web requests of Web Server Gateway Interface (WSGI)- and Asynchronous Server Gateway Interface (ASGI)-based applications for supported frameworks and modules. For a more detailed analysis, you can utilize the custom instrumentation APIs. This enables you to analyze specific transactions or code blocks in your applications.
This documentation covers APIs, including their functionality and syntax.
Monitor transactions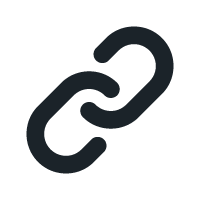
By default, the agent automatically captures and displays incoming web requests in the Transactions > Web tab. However, the agent does not monitor other types of client-server communications, such as socket connections, scheduler calls, threading methods, and asynchronous calls. To track these transactions, you can use the provided API.
Similarly, background transactions are not monitored by default, but you can utilize the API to instrument and monitor them.
This API customizes transaction names or excludes specific transactions from monitoring as needed, providing flexibility and control over your application's monitoring capabilities.
API to capture background transactions using the decorator method
Syntax:
#decorator
apminsight.background_transaction(name="transaction_name")
Example:
import apminsight
@apminsight.background_transaction(name="transaction_name")
def custom_method(*args, **kwargs):
# func definition
#
# func definition
Capturing transactions using context-based classes
Syntax:
while apminsight.TransactionContext(name="txn_name",tracker_name="root_traker_name"):
Example:
import apminsight
while apminsight.TransactionContext( name="transction_name", tracker_name= "root_racker_name"):
# code logic
# code logic
Capture background transactions using start_background_transaction API
Syntax:
apminsight.start_background_transaction(name="transaction_name")
Example:
def custom_method(*args, **kwargs):
# code logic
apminsight.start_background_transaction( name="custom_txn_name")
# code logic
# code logic
apminsight.end_transaction()
# code logic
The start_background_transaction API should be followed by the end_transaction API call to close the transaction properly.
Customize the transaction name
You can use this API to modify the name of the current transaction.
Syntax:
apminsight.customize_transaction_name("txn_name")
Ignore a transaction
You can use this API to remove a transaction from monitoring data once the current transaction has finished.
Syntax:
apminsight.ignore_transaction()
Custom tracker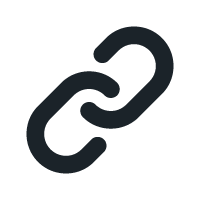
By default, the agent automatically captures framework- and module-related methods. To monitor user-defined classes and methods, they must be instrumented using the provided API. This data can be viewed in the Traces tab. Additionally, any transaction involving database operations called within the instrumented class or method can be viewed in the Database tab.
API for custom tracker using the decorator method
Syntax:
apminsight.custom_tracker(name="tracker_name")
Example:
import apminsight
import sqlite3
@apminsight.custom_tracker(name="updated_query")
def get_query_with_args(query_string, *args, **kwargs):
# valid query check
# parametrizing the query
return query_string
@apminsight.background_transaction(name="do_query")
def perform_query_operation(*args, **kwargs):
sqliteConnection = sqlite3.connect('sql.db')
query = get_query_with_args(*args, **kwargs)
cursor = sqliteConnection.cursor()
cursor.execute(query)
Tracking blocks of code using context-based classes
Syntax:
apminsight.TrackerContext(name="tracker_name")
Example:
import apminsight
import sqlite3
def get_query_with_args(query_string, *args, **kwargs):
# valid query check
# parametrizing the query
return query_string
@apminsight.background_transaction(name="do_query")
def perform_query_operation(*args, **kwargs):
sqliteConnection = sqlite3.connect('sql.db')
query = ""
while apminsight.TrackerContext( name="get_query_string"):
query = get_query_with_args(*args, **kwargs)
cursor = sqliteConnection.cursor()
cursor.execute(query)
Track custom parameters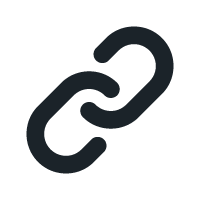
To give contextual meaning to traces, you can add additional parameters that can help you identify the context of the transaction traces.
Contextual metrics can be anything, such as a session ID, user ID, or specific method parameters, that can help you identify the details of a transaction trace.
You can add a maximum of 10 parameters to a transaction trace, which can be viewed by navigating to Traces, clicking the trace, and going to the Summary tab.
Syntax:
apminsight.add_custom_param("key", "value");
Example:
import apminsight
@apminsight.background_transaction(name="custom_params_txn")
def custom_method(*args, **kwargs):
apminsight.add_custom_param("sampling_response",0.12)
apminsight.add_custom_param("Agent","apminsight")
Track custom exceptions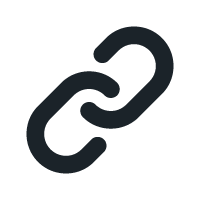
The agent generally captures caught and uncaught exceptions. This API is useful if you want to capture exceptions from un-instrumented blocks of code.
Syntax:
apminsight.add_custom_exception(err:Exception)
Example:
import apminsight
@apminsight.background_transaction(name="custom_exception")
def square_root(n):
try:
return n ** 0.5
except ValueError as exc:
apminsight.add_custom_exception(ValueError('n must be greater then 0'))
raise exc